Why Moleculer Framework?

Moleculer is a javascript framework for creating microservices, write your app as a monolithic application that will work as independent services, and the big difference will be the deployment step.
Using Moleculer is very easy, the architecture is simple, the learning curve is fast, and with good documentation, different from other frameworks.

Moleculer uses API Gateway as the entry point when using REST, and from there, you call the other microservices.
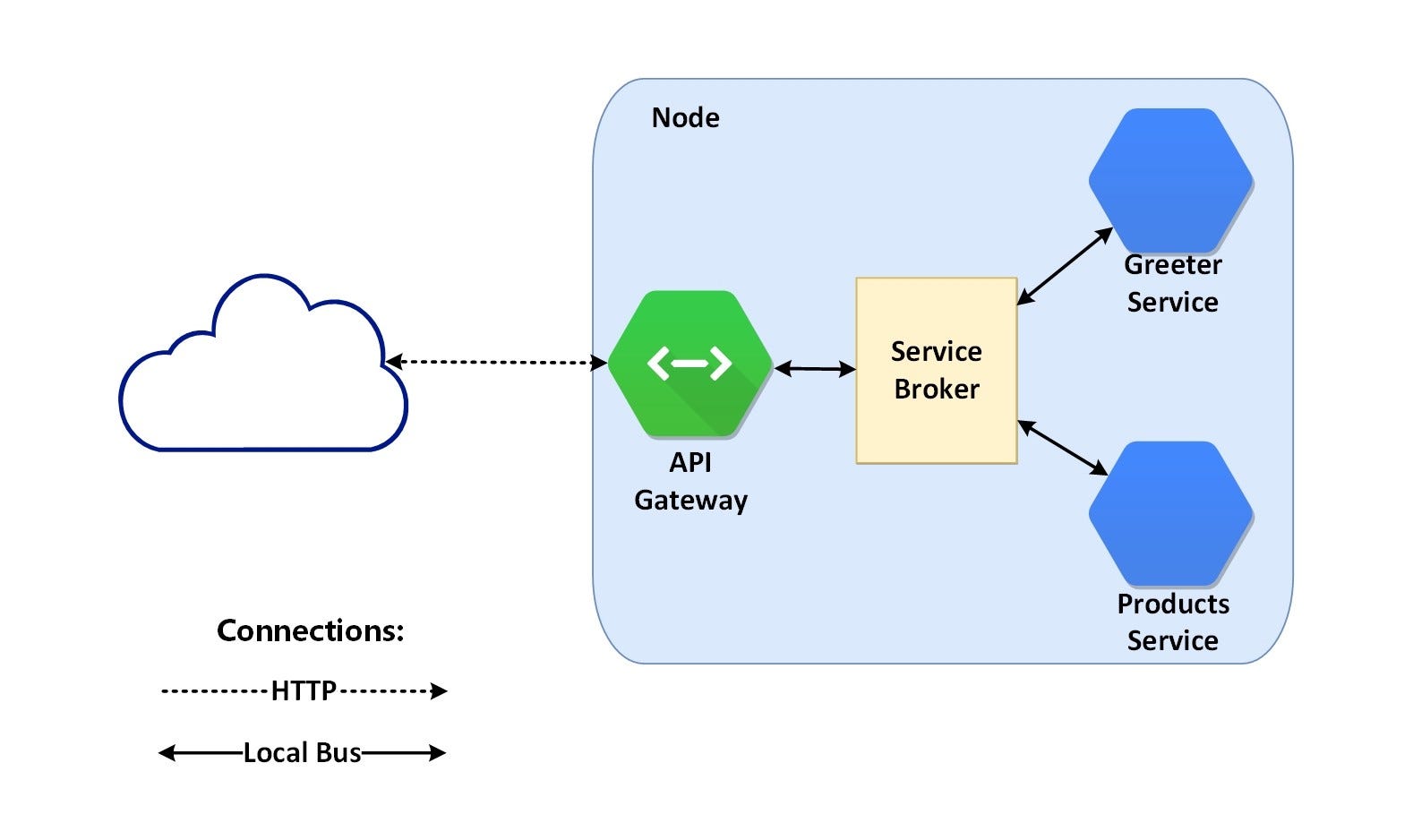
Let’s see a benchmark comparing with other frameworks:


Check the results on your computer! Just clone this repo and run npm install && npm start
.
Check out our benchmark results.
Moleculer has excellent documentation, where you can learn the concepts, the transport layer for inter-service communication like (TCP, NATS, MQTT, Redis, NATS Streaming, Kafka, AMQP 0.9, AMQP 1.0) and all the other steps to get it up and running.
Security
Moleculer offers a secure method for your keys and tokens using $secureSettings from within your service.
// mail.service.js
module.exports = {
name: "mailer",
settings: {
$secureSettings: ["transport.auth.user", "transport.auth.pass"],from: "sender@moleculer.services",
transport: {
service: 'gmail',
auth: {
user: 'gmail.user@gmail.com',
pass: 'yourpass'
}
}
}
// ...
};
Mixins
One very interesting resource from Moleculer is the mixins. It distributes reusable functionalities for all services in one single place, but available at any time from any service.
// api.service.js
const ApiGwService = require("moleculer-web");module.exports = {
name: "api",
mixins: [ApiGwService]
settings: {
// Change port setting
port: 8080
},
actions: {
myAction() {
// Add a new action to apiGwService service
}
}
}
Events
For asynchronous communication when ACK is not needed, you can use events to call some action using fire and forget. Useful for custom actions or displaying logs as an example.
// report.service.js
module.exports = {
name: "report",events: {
// Subscribe to "user.created" event
"user.created"(ctx) {
this.logger.info("User created:", ctx.params);
// Do something
},// Subscribe to all "user.*" events
"user.*"(ctx) {
console.log("Payload:", ctx.params);
console.log("Sender:", ctx.nodeID);
console.log("Metadata:", ctx.meta);
console.log("The called event name:", ctx.eventName);
}// Subscribe to a local event
"$node.connected"(ctx) {
this.logger.info(`Node '${ctx.params.id}' is connected!`);
}
}
};
Lifecycle Events
Moleculer has a simple lifecycle as shown below.
// www.service.js
module.exports = {
name: "www",
actions: {...},
events: {...},
methods: {...},// Fired when the service instance created (with `broker.loadService` or `broker.createService`)
created() {
},// Fired when broker starts this service (in broker.start())
started() {
},// Fired when broker stops this service (in `broker.stop()`)
stopped() {
}
};
Actions
The action calls represent a remote-procedure-call (RPC), but for those familiar with REST servers, it brings the concept of request/response to make it easy to work with.

Note: The default values are
null
(meanspublished
) due to backward compatibility. You can also use the visibility parameter to restrict access to actions.
module.exports = {
name: "posts",
actions: {
// It's published by default
find(ctx) {},
clean: {
// Callable only via `this.actions.clean`
visibility: "private",
handler(ctx) {}
}
},
methods: {
cleanEntities() {
// Call the action directly
return this.actions.clean();
}
}
}
When using actions, you can call any service or event using afterHook or beforeHook, it is very handy when you need to process some custom step to change the request or the response for example. Read more here.
Local Storage & Hooks
With Moleculer, you can save data in the request as params for using during its lifetime with the help of hooks as well.
module.exports = {
name: "user",hooks: {
before: {
async get(ctx) {
const entity = await this.findEntity(ctx.params.id);
ctx.locals.entity = entity;
}
}
},actions: {
get: {
params: {
id: "number"
},
handler(ctx) {
this.logger.info("Entity", ctx.locals.entity);
}
}
}
}
Balanced events
The event listeners are arranged as logical groups. It means that only one listener is triggered in every group call, balancing the requests under the hood. Read more about events here.

It also has support for typescript. Here is a skeleton project to get started: https://github.com/moleculerjs/moleculer-template-project-typescript
Finally, it wouldn't be possible to list all the advantages of using Moleculer in one article only and this is just to get started. Keep one eye on our channel for more.
See you next time
Telegram Group
https://t.me/moleculer_en
References: